When I first heard about Kevin Geary’s EtchWP product announcement, it got me thinking. WordPress has strayed pretty far from its roots, and I believe his ideas align with what I’ve been hoping for—a more streamlined, developer-friendly WordPress experience that focuses on PHP and drastically reduces the current obsession with JavaScript-heavy workflows.
Now, I’ll be honest: I’m not fully on board with the tone of the discourse surrounding these discussions. Still, I can’t help but be excited about any disruption that can cause a course correction for WordPress.
The Problem with WordPress Development Today
Developing WordPress used to be fun. You could quickly build custom themes, create layouts, and make something unique with just a bit of PHP knowledge. But today? That simplicity is long gone. This been been mentioned over and over again, and the last thing I want is this post to be about this, all I want to say about is that in my subjective opinion the developer & agency experience is what ultimately makes any platform successful, and I think WordPress has long lost that key aspect of working with the platform.
Let’s me share my thoughts about what I hope the future of WordPress development looks like, being fully aware of the massive implications of what I’m about to write below.
Laravel & Livewire: A Breath of Fresh Air
For those who haven’t worked with Laravel, here’s a quick rundown. Laravel offers a framework where developers can quickly generate interactive components using just PHP, all while utilizing Blade templates—a clean, intuitive way to manage front-end views. Blade is a simple but powerful templating engine, and it has a minimal learning curve for anyone who’s ever built a WordPress theme.
Livewire then takes this a step further. It allows developers to create dynamic, reactive components using only PHP—no JavaScript required. Think about that for a second: you can build complex, interactive components without having to toggle back and forth between PHP and JavaScript. Everything stays seamless and in the same context. This means faster development and a far less fragmented workflow.
Applying This Approach to WordPress
Now, imagine WordPress adopting a similar approach. Custom fields, blocks, settings pages, and templates could all be registered and managed through a consistent, opinionated framework. We could ditch the bloated JavaScript approach in favor of something more streamlined—something that uses PHP as the primary driver again, but with the added benefits of modern interactivity.
I’ve been pretty vocal about this on Twitter: it’s not fun to develop in WordPress anymore. The excitement of quickly spinning up a theme or customizing a layout has been replaced with a maze of JS libraries, dependencies, and constant build processes. That needs to change.
Roots Acorn
Roots Acorn is by far one of the most innovative projects I’ve had the pleasure to work with. Because it does (almost) everything I outlined above. You can use Livewire, Laravel routing, blade templating and so much more inside WordPress. For example take this layout:
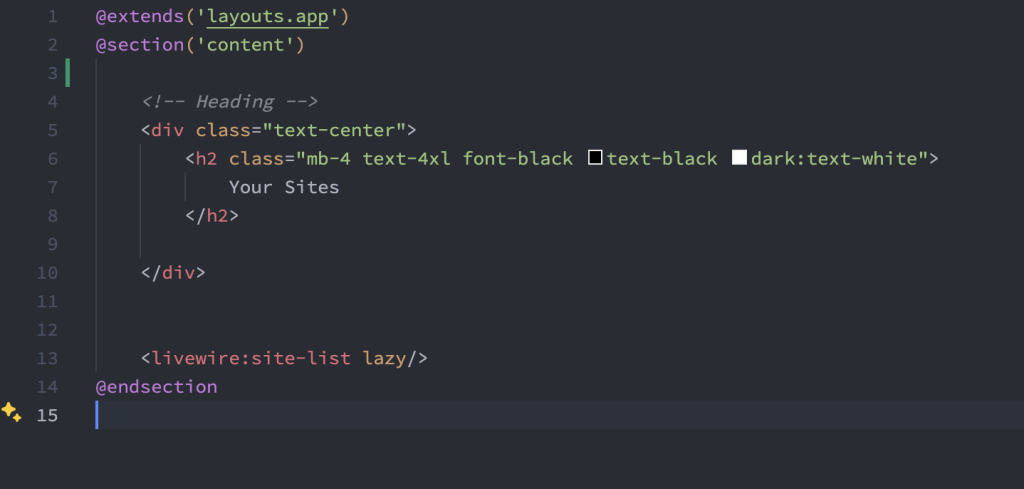
Renders the following in our upcoming “Client Portal Builder” for Dollie. All of this is also fully compatible with WordPress, meaning you can render livewire components easily using shortcodes, custom functions, or inside page builders via custom widgets.
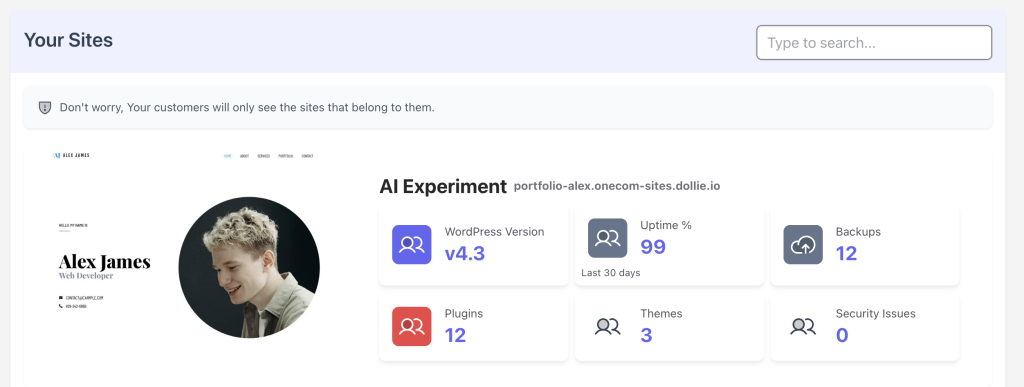
Looking at the code you can guess a little bit what’s going on in here. The sites component is lazy loaded, so it does not blocks the rendering of the page. See the “lazy” attribute.
When the component is rendered you can make an external API request, or do a heavy database query without slowing down the page. Now what happens if you want to search the sites? You type, Livewire receives the requests and updates the component instantly. Here’s ALL the code for this entire component.
<?php
namespace DollieHubApp\Livewire;
use Illuminate\View\View;
use Livewire\Attributes\Url;
use Livewire\Component;
/**
* Class SiteList
*
* The SiteList class is a component used to render the client websites and their associated statistics in a list format.
* It extends the Component class.
*/
class SiteList extends Component
{
#[Url]
public string $query = ''; // Search query
protected $listeners = [
'refreshParentComponent' => '$refresh',
];
public function placeholder(array $params = [])
{
return view('components.helpers.site-loading', $params);
}
/**
* Create an array of widgets to pass to the view.
*/
public function createWidgets($site)
{
//Widget Settings
}
/**
* Render the component.
*
* @return View
*/
public function render()
{
// Get the search query
$searchQuery = $this->query;
// Get all sites based on user permissions and the search query
if (dollie()->get_user()->can_view_all_sites()) {
$sites = dollie()->get_containers($searchQuery);
} else {
$sites = dollie()->get_customer_containers(null, $searchQuery);
}
// Pass the sites and stats to the view
return view('livewire.site-list', [
'sites' => $sites,
]);
}
}
Disruption is needed
Here’s where I hope Kevin Geary’s vision (and others in the community) could come into play. We need a framework like Acorn, or EtchWP that gets tractions and gets adopted that provides an alternative to what we have current.y
Something that combines the simplicity of PHP with the power of modern development, without relying so heavily on JavaScript. Something like Laravel and Livewire but built into WordPress—making it easier to create components, register blocks, and build layouts, all with a consistent design system and a streamlined editor.
I can imagine a future where there’s an open marketplace for new components, allowing designers and developers to quickly add functionality or build their own. There’s no need to throw out the good parts of WordPress, but it’s not too late to pivot.
It’s Not Too Late for WordPress to Change
To be clear, I don’t think it’s too late for WordPress. Yes, the Gutenberg direction has had its issues, but there’s still a chance to course-correct. WordPress has an opportunity to simplify development again, to create a faster workflow with far fewer dependencies on JavaScript, and to make the whole ecosystem more maintainable for the long term. This could be the start of a more productive, consistent, and enjoyable way to build on WordPress.
And if Kevin’s not working on exactly this, there are still tools out there—like Roots Acorn—that can give you the workflow I’m talking about. So, it’s not like we’re starting from scratch.
Pseudo-Code: What Could This Look Like?
If WordPress were to adopt something more like Livewire, here’s how I imagine the code would look when registering a block or component, with PHP and Blade doing the heavy lifting.
1. Registering a Blade Component (Block) in WordPress:
Register a block with Blade as the view template<br>function register_custom_block() {<br> register_block_type('my-plugin/custom-block', [<br> 'render_callback' => function( $attributes ) {<br> return view('components.custom-block', ['attributes' => $attributes])->render();<br> }<br> ]);<br>}<br>add_action('init', 'register_custom_block');<br>
2. Blade Template for the Block (components/custom-block.blade.php):
<div class="custom-block"><br> <h2>{{ $attributes['title'] }}</h2><br> <p>{{ $attributes['content'] }}</p><br></div><br>
3. Dynamic Interaction with Livewire (Alpine-like component):
// Register a Livewire-style dynamic component<br>function register_dynamic_component() {<br> register_block_type('my-plugin/dynamic-component', [<br> 'render_callback' => function( $attributes ) {<br> return view('components.dynamic-component', ['attributes' => $attributes])->render();<br> }<br> ]);<br>}<br>add_action('init', 'register_dynamic_component');<br>
4. Blade Template with Dynamic Behavior (components/dynamic-component.blade.php):
<div><br> <button wire:click="increment">Click me</button><br> <span>{{ $count }}</span><br></div><br>
5. Livewire Component (DynamicComponent.php):
use Livewire\Component;<br><br>class DynamicComponent extends Component {<br> public $count = 0;<br><br> public function increment() {<br> $this->count++;<br> }<br><br> public function render() {<br> return view('livewire.dynamic-component');<br> }<br>}<br>
Final Thoughts
WordPress is at a crossroads. We can either continue down the JavaScript-heavy path that’s frustrated so many of us, or we can embrace a new, more streamlined approach that brings the fun and simplicity back to development. I, for one, am rooting for the latter.
Thanks for coming to my TED Talk 😉.